Me:
Can you please create the following:
1. An html login page that asks for a username and password. The form should submit to a login.php page.
2. A login.php page. This should connect to a mysql database table. The password should not be stored in plaintext. On successful login, it should create a cookie and redirect to a welcome.php page. If the password is incorrect, it should print a “login failed” message.
3. SQL to create the mysql database table for username and passwords that login.php references. It should contain a user_id column, which will act as the primary key.
4. a welcome.php page. It should print “You have successfully logged in” if the browser has the cookie from a successful login, otherwise it should print a “You are not logged in” message.
ChatGPT:
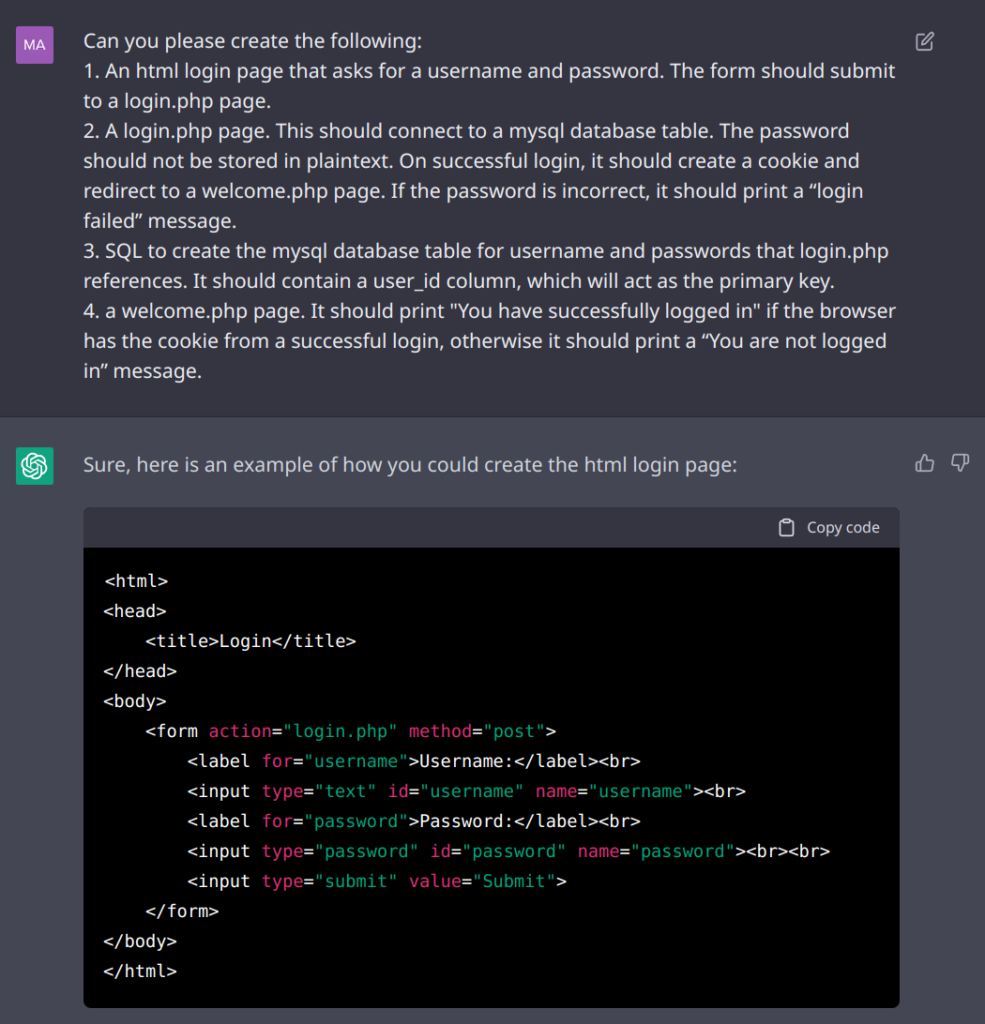
![This is an example of the login.php page that connects to a mysql database table and checks the entered password:
Copy code
<?php
$username = $_POST["username"];
$password = $_POST["password"];
//connect to mysql database
$servername = "localhost";
$dbUsername = "myusername";
$dbPassword = "mypassword";
$dbName = "mydatabase";
$conn = new mysqli($servername, $dbUsername, $dbPassword, $dbName);
//check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
//query the database for the entered username
$sql = "SELECT * FROM users WHERE username = '$username'";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
//if the username is found, check the password
if ($result->num_rows > 0) {
//hash the entered password and compare it to the stored password hash
if (password_verify($password, $row["password"])) {
//if the password is correct, create a cookie and redirect to the welcome page
setcookie("username", $username, time() + (86400 * 30), "/"); //86400 = 1 day
header("Location: welcome.php");
} else {
//if the password is incorrect, print a login failed message
echo "Login failed";
}
} else {
//if the username is not found, print a login failed message
echo "Login failed";
}
$conn->close();
?>](https://ai.mattjung.net/wp-content/uploads/2022/12/2022-12-06-21-59-34-581x1024.png)
![Here is an example of SQL to create the mysql database table for the username and passwords that the login.php page references:
Copy code
CREATE TABLE users (
user_id INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
password VARCHAR(255) NOT NULL
);
And finally, here is an example of the welcome.php page that checks for a cookie and prints the appropriate message:
Copy code
<html>
<head>
<title>Welcome</title>
</head>
<body>
<?php
if (isset($_COOKIE["username"])) {
echo "You have successfully logged in";
} else {
echo "You are not logged in";
}
?>
</body>
</html>](https://ai.mattjung.net/wp-content/uploads/2022/12/2022-12-06-21-59-45-900x1024.png)
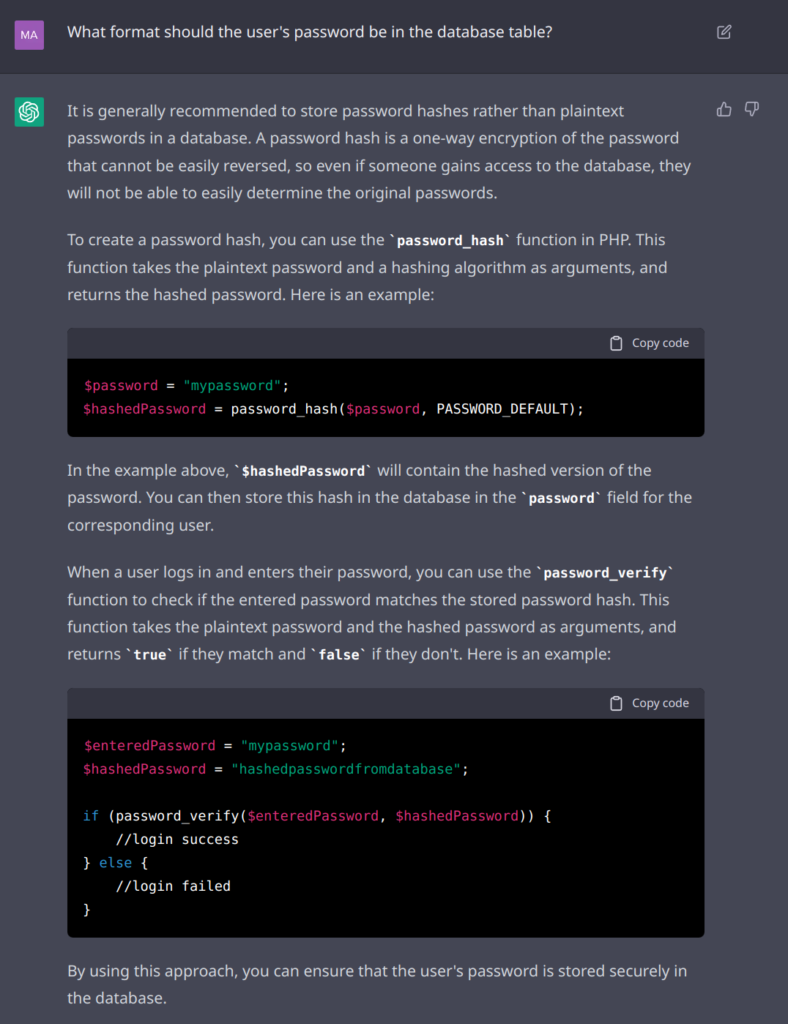
Conclusion
It’s impressive that this does work:
https://ai.mattjung.net/login.html
matt
GPtest1237H
The only manual steps I needed to do were:
- Create the database
- Create the database user
- Add grants to the database user
- Insert a user into the database table
- Populate database connection info into login.php
It also had some shortcomings. In earlier attempts:
- It did not hash the password in the database. It stored the password in the database as plaintext.
- It did not have a primary key in the database table.
It’s not a bad start but the lack of basic security practices is a concern. If someone were to create their own application that ultimately stores sensitive data with ChatGPT, it seems like there is a significant risk that the data could be easily leaked by an attacker under the right circumstances.
Leave a Reply